How Do You Handle API Versioning In A MERN Stack Project?
Learn effective API versioning strategies in MERN Stack projects to ensure seamless updates, backward compatibility, and maintainable APIs.
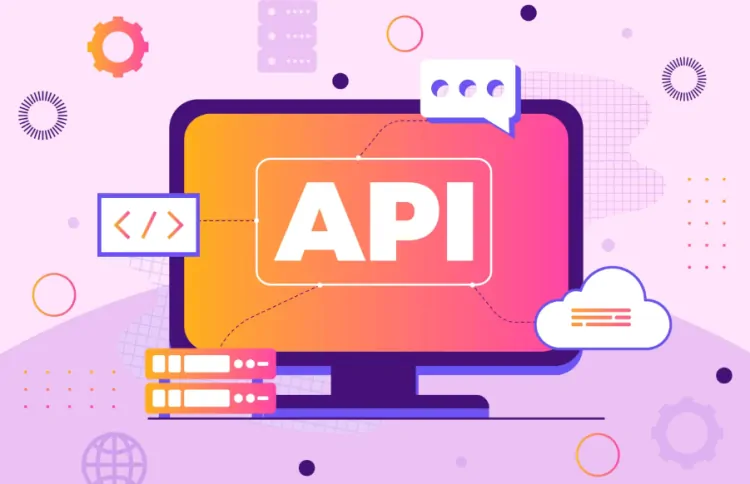
Introduction
API versioning is essential for maintaining backward compatibility while evolving your application. In a MERN stack project (MongoDB, Express.js, React, Node.js), it ensures seamless integration of new features without disrupting existing clients. By managing different API versions effectively, developers can support incremental updates, isolate errors, and enhance system flexibility. Consider joining the MERN Stack Certification course for the best learning opportunities. This guide explores various versioning strategies like URI paths, headers, and query parameters to ensure robust and scalable API development.
Steps To Handle API Versioning In A MERN Stack Project
API versioning is a crucial practice in software development to ensure backward compatibility while introducing changes or enhancements to your APIs. In a MERN stack project (MongoDB, Express.js, React, Node.js), versioning ensures that existing clients can still use older versions of the API without disruption, even as you implement new features or deprecate old ones.
Here's how to effectively handle API versioning in a MERN stack project:
Why API Versioning is Important?
· Backward Compatibility: Ensures existing consumers of the API are not broken by new updates.
· Incremental Updates: Allows developers to iterate on APIs without rushing to migrate every client at once.
· Error Isolation: Helps in debugging by segregating issues specific to a version.
Methods for API Versioning
1. URI Versioning (Preferred)
Add the version number in the URI path, e.g., /api/v1/resource.
Example:
“const express = require('express');
const app = express();
app.get('/api/v1/users', (req, res) => {
res.send({ message: 'API v1: List of users' });
});
app.get('/api/v2/users', (req, res) => {
res.send({ message: 'API v2: List of users with more details' });
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});”
2. Header Versioning
Include the version in the request headers. Check the Mern Stack Course in Hyderabad for more information.
“app.get('/api/users', (req, res) => {
const version = req.headers['api-version'];
if (version === '1.0') {
res.send({ message: 'API v1: List of users' });
} else if (version === '2.0') {
res.send({ message: 'API v2: List of users with more details' });
} else {
res.status(400).send({ error: 'Unsupported API version' });
}
});”
3. Query Parameter Versioning
Pass the version as a query parameter.
Example:
“app.get('/api/users', (req, res) => {
const version = req.query.version;
if (version === '1') {
res.send({ message: 'API v1: List of users' });
} else if (version === '2') {
res.send({ message: 'API v2: List of users with more details' });
} else {
res.status(400).send({ error: 'Unsupported API version' });
}
});”
4. Content Negotiation
Use the Accept header to specify the version in MIME type.
Example:
“app.get('/api/users', (req, res) => {
const acceptHeader = req.headers['accept'];
if (acceptHeader.includes('application/vnd.api.v1+json')) {
res.send({ message: 'API v1: List of users' });
} else if (acceptHeader.includes('application/vnd.api.v2+json')) {
res.send({ message: 'API v2: List of users with more details' });
} else {
res.status(400).send({ error: 'Unsupported API version' });
}
});”
Best Practices
· Use Semantic Versioning: Follow a pattern like v1.0, v2.0 to make changes predictable.
· Deprecation Strategy: Communicate with API consumers about deprecated versions and provide a sunset timeline.
· Modular Routing: Organize routes by version to keep your codebase maintainable.
“const v1Routes = require('./routes/v1');
const v2Routes = require('./routes/v2');
app.use('/api/v1', v1Routes);
app.use('/api/v2', v2Routes);”
· Testing: Ensure every version is independently tested for functionality and performance.
Conclusion
API versioning in a MERN stack project ensures smooth evolution and scalability of your application. Many Mern Stack Developer Interview Questions include questions on API versioning, thereby, making it an important topic for MERN professionals. Among the methods available, URI versioning is the most common and developer-friendly. Combining a clear deprecation strategy and modular architecture will make version management efficient, keeping both developers and clients happy.
What's Your Reaction?






